Tutorial for serving static gzip with webpack module bundler and nginx server. When you would use nginx directly to compress the output, it would do it on the run and with some load increase the cpu usage. It's better to compress your huge project a single time and then serve it when possible. So webpack creates the compressed files and nginx servers gzip instead of the original when possible.
Webpack
Install webpack compression plugin and add it to dev dependencies.Edit your webpack production config file to zip the files on compilation. The config has some basic extensions of javascript, styles and fonts to compress only where it counts. Threshold limits the file size that is valid for compression and minRatio defines the minimum compression ratio which the zip file is accepted, everything above that is not compressed to get the optimal page load speed.Source code viewer
npm install --save-dev compression-webpack-pluginProgramming Language: Bash
Source code viewer
const CompressionPlugin = require('compression-webpack-plugin'); module.exports = { plugins: [ new CompressionPlugin({ asset: '[path].gz[query]', algorithm: 'gzip', test: /\.(js|css|ttf|svg|eot)$/, threshold: 10240, minRatio: 0.8, }), ] }Programming Language: ECMAScript
Nginx
Make sure you have http_gzip_static_module with "nginx -V". If you don't have the module included you might need a custom compiled nginx for an example Ubuntu server nginx packages have it included by default. Add to nginx configuration.Source code viewer
# Enable ngx_http_gzip_static_module for serving compressed files when possible. gzip_static on;Programming Language: nginx
Chrome
Validate that your styles get compressed by "Content-Encoding: gzip" response header.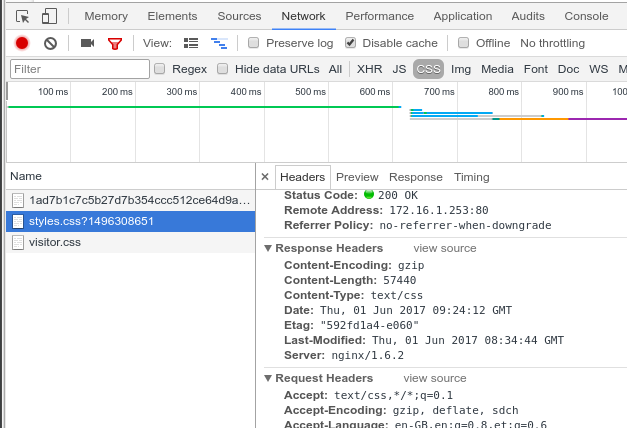